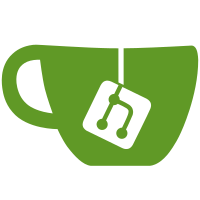
better path going forward to handling upgrades. Old syntax files will stick around for compatibility purposes. Old syntax can be parsed into valid syntax trees via the old syntax (.peg) files, and then old syntax trees should be valid and can be upgraded via the normal code path. This change has lots of improvements to Nomsu codegen too.
64 lines
2.2 KiB
Plaintext
64 lines
2.2 KiB
Plaintext
#!/usr/bin/env nomsu -V1
|
|
#
|
|
This file contains some definitions of text escape sequences, including ANSI console
|
|
color codes.
|
|
|
|
use "core/metaprogramming.nom"
|
|
|
|
# Text functions
|
|
action [%texts joined with %glue]
|
|
lua> ".."
|
|
local text_bits = {}
|
|
for i,bit in ipairs(\%texts) do text_bits[i] = stringify(bit) end
|
|
return table.concat(text_bits, \%glue)
|
|
parse [joined %texts, %texts joined] as: %texts joined with ""
|
|
|
|
compile [capitalized %text, %text capitalized] to
|
|
Lua value "((\(%text as lua expr)):gsub('%l', string.upper, 1))"
|
|
|
|
compile [%text with %sub instead of %patt, %text with %patt replaced by %sub, %text s/%patt / %sub] to
|
|
Lua value "((\(%text as lua expr)):gsub(\(%patt as lua expr), \(%sub as lua expr)))"
|
|
|
|
action [lines in %text, lines of %text]
|
|
lua> ".."
|
|
local result = list{}
|
|
for line in (\%text):gmatch('[^\n]+') do
|
|
result[#result+1] = line
|
|
end
|
|
return result
|
|
|
|
compile [for %match where %text matches %patt %body] to
|
|
Lua ".."
|
|
for \(%match as lua expr) in \(%text as lua expr):gmatch(\(%patt as lua expr)) do
|
|
\(%body as lua statements)
|
|
\(compile as: === next %match ===)
|
|
end
|
|
\(compile as: === stop %match ===)
|
|
|
|
compile [%expr for %match where %text matches %patt] to
|
|
Lua value ".."
|
|
(function()
|
|
local ret = list{}
|
|
for \(%match as lua expr) in \(%text as lua expr):gmatch(\(%patt as lua expr)) do
|
|
ret[#ret+1] = \(%expr as lua statements)
|
|
end
|
|
return ret
|
|
end)()
|
|
|
|
compile [%text matches %pattern] to
|
|
Lua value "(\(%text as lua expr):match(\(%pattern as lua expr)) and true or false)"
|
|
|
|
# Text literals
|
|
lua> ".."
|
|
do
|
|
local escapes = {
|
|
nl="\\\\n", newline="\\\\n", tab="\\\\t", bell="\\\\a", cr="\\\\r", ["carriage return"]="\\\\r",
|
|
backspace="\\\\b", ["form feed"]="\\\\f", formfeed="\\\\f", ["vertical tab"]="\\\\v",
|
|
};
|
|
for name, e in pairs(escapes) do
|
|
local lua = "'"..e.."'"
|
|
nomsu.COMPILE_ACTIONS[name] = (function(nomsu, tree) return LuaCode.Value(tree.source, lua) end)
|
|
end
|
|
end
|
|
|