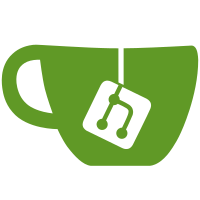
better path going forward to handling upgrades. Old syntax files will stick around for compatibility purposes. Old syntax can be parsed into valid syntax trees via the old syntax (.peg) files, and then old syntax trees should be valid and can be upgraded via the normal code path. This change has lots of improvements to Nomsu codegen too.
70 lines
2.5 KiB
Plaintext
70 lines
2.5 KiB
Plaintext
#!/usr/bin/env nomsu -V1
|
|
#
|
|
This file contains basic error reporting code
|
|
|
|
use "core/metaprogramming.nom"
|
|
|
|
compile [traceback] to: Lua value "debug.traceback()"
|
|
compile [traceback %] to: Lua value "debug.traceback('', \(% as lua expr))"
|
|
compile [barf] to: Lua "error(nil, 0);"
|
|
compile [barf %msg] to: Lua "error(\(%msg as lua expr), 0);"
|
|
compile [assume %condition] to
|
|
lua> "local \%assumption = 'Assumption failed: '..tostring(nomsu:tree_to_nomsu(\%condition))"
|
|
return
|
|
Lua ".."
|
|
if not \(%condition as lua expr) then
|
|
error(\(quote "\%assumption"), 0)
|
|
end
|
|
|
|
compile [assume %condition or barf %message] to
|
|
Lua ".."
|
|
if not \(%condition as lua expr) then
|
|
error(\(%message as lua expr), 0)
|
|
end
|
|
|
|
# Try/except
|
|
compile [..]
|
|
try %action and if it succeeds %success or if it barfs %msg %fallback
|
|
try %action and if it barfs %msg %fallback or if it succeeds %success
|
|
..to
|
|
Lua ".."
|
|
do
|
|
local fell_through = false
|
|
local err, erred = nil, false
|
|
local ok, ret = xpcall(function()
|
|
\(%action as lua statements)
|
|
fell_through = true
|
|
end, function(\(%msg as lua expr))
|
|
local ok, ret = pcall(function()
|
|
\(%fallback as lua statements)
|
|
end)
|
|
if not ok then err, erred = ret, true end
|
|
end)
|
|
if ok then
|
|
\(%success as lua statements)
|
|
if not fell_through then
|
|
return ret
|
|
end
|
|
elseif erred then
|
|
error(err, 0)
|
|
end
|
|
end
|
|
|
|
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
parse [..]
|
|
try %action and if it succeeds %success or if it barfs %fallback
|
|
try %action and if it barfs %fallback or if it succeeds %success
|
|
..as: try %action and if it succeeds %success or if it barfs (=lua "") %fallback
|
|
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
parse [try %action] as
|
|
try %action and if it succeeds: do nothing
|
|
..or if it barfs: do nothing
|
|
parse [try %action and if it barfs %fallback] as
|
|
try %action and if it succeeds: do nothing
|
|
..or if it barfs %fallback
|
|
parse [try %action and if it barfs %msg %fallback] as
|
|
try %action and if it succeeds: do nothing
|
|
..or if it barfs %msg %fallback
|
|
parse [try %action and if it succeeds %success] as
|
|
try %action and if it succeeds %success or if it barfs: do nothing
|