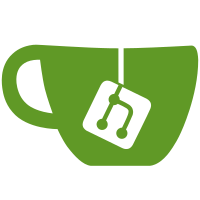
better path going forward to handling upgrades. Old syntax files will stick around for compatibility purposes. Old syntax can be parsed into valid syntax trees via the old syntax (.peg) files, and then old syntax trees should be valid and can be upgraded via the normal code path. This change has lots of improvements to Nomsu codegen too.
241 lines
9.5 KiB
Plaintext
241 lines
9.5 KiB
Plaintext
#!/usr/bin/env nomsu -V1
|
|
#
|
|
This File contains actions for making actions and compile-time actions and some helper
|
|
functions to make that easier.
|
|
|
|
lua> "NOMSU_CORE_VERSION = 2"
|
|
|
|
lua> ".."
|
|
nomsu.COMPILE_ACTIONS["% -> %"] = function(nomsu, tree, \%args, \%body)
|
|
local lua = LuaCode.Value(tree.source, "function(")
|
|
if AST.is_syntax_tree(\%args, "Action") then \%args = \%args:get_args() end
|
|
local lua_args = table.map(\%args, function(a) return AST.is_syntax_tree(a) and tostring(nomsu:compile(a)) or a end)
|
|
lua:concat_append(lua_args, ", ")
|
|
local body_lua = AST.is_syntax_tree(\%body) and nomsu:compile(\%body):as_statements("return ") or \%body
|
|
body_lua:remove_free_vars(lua_args)
|
|
body_lua:declare_locals()
|
|
lua:append(")\n ", body_lua, "\nend")
|
|
return lua
|
|
end
|
|
|
|
lua> ".."
|
|
nomsu.COMPILE_ACTIONS["compile as %"] = function(nomsu, tree, \%action)
|
|
local lua = LuaCode.Value(tree.source, "nomsu.COMPILE_ACTIONS[", repr(\%action.stub), "](")
|
|
local lua_args = table.map(\%action:get_args(), function(a) return nomsu:compile(a) end)
|
|
table.insert(lua_args, 1, "nomsu")
|
|
table.insert(lua_args, 2, "tree")
|
|
lua:concat_append(lua_args, ", ")
|
|
lua:append(")")
|
|
return lua
|
|
end
|
|
|
|
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
|
|
lua> ".."
|
|
nomsu.COMPILE_ACTIONS["compile % to %"] = function(nomsu, tree, \%actions, \%body)
|
|
local \%args = {"nomsu", "tree", unpack(table.map(\%actions[1]:get_args(), function(a) return tostring(nomsu:compile(a)) end))}
|
|
local lua = LuaCode(tree.source, "nomsu.COMPILE_ACTIONS[", repr(\%actions[1].stub),
|
|
"] = ", \(compile as: %args -> %body))
|
|
for i=2,#\%actions do
|
|
local alias = \%actions[i]
|
|
local \%alias_args = {"nomsu", "tree", unpack(table.map(alias:get_args(), function(a) return tostring(nomsu:compile(a)) end))}
|
|
lua:append("\nnomsu.COMPILE_ACTIONS[", repr(alias.stub), "] = ")
|
|
if utils.equivalent(\%args, \%alias_args) then
|
|
lua:append("nomsu.COMPILE_ACTIONS[", repr(\%actions[1].stub), "]")
|
|
else
|
|
lua:append("function(")
|
|
lua:concat_append(\%alias_args, ", ")
|
|
lua:append(")\n return nomsu.COMPILE_ACTIONS[", repr(\%actions[1].stub), "](")
|
|
lua:concat_append(\%args, ", ")
|
|
lua:append(")\nend")
|
|
end
|
|
end
|
|
return lua
|
|
end
|
|
|
|
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
|
|
compile [call %fn with %args] to
|
|
lua> ".."
|
|
local lua = LuaCode.Value(tree.source, nomsu:compile(\%fn), "(")
|
|
lua:concat_append(table.map(\%args, function(a) return nomsu:compile(a) end), ", ")
|
|
lua:append(")")
|
|
return lua
|
|
|
|
compile [local action %actions %body] to
|
|
lua> ".."
|
|
local fn_name = "A"..string.as_lua_id(\%actions[1].stub)
|
|
local \%args = table.map(\%actions[1]:get_args(), function(a) return tostring(nomsu:compile(a)) end)
|
|
local lua = LuaCode(tree.source, fn_name, " = ", \(compile as: %args -> %body))
|
|
lua:add_free_vars({fn_name})
|
|
for i=2,#\%actions do
|
|
local alias = \%actions[i]
|
|
local alias_name = "A"..string.as_lua_id(alias.stub)
|
|
lua:add_free_vars({alias_name})
|
|
local \%alias_args = table.map(alias:get_args(), function(a) return tostring(nomsu:compile(a)) end)
|
|
lua:append("\n", alias_name, " = ")
|
|
if utils.equivalent(\%args, \%alias_args) then
|
|
lua:append(fn_name)
|
|
else
|
|
lua:append("function(")
|
|
lua:concat_append(\%alias_args, ", ")
|
|
lua:append(")\n return ", fn_name, "(")
|
|
lua:concat_append(\%args, ", ")
|
|
lua:append(")\nend")
|
|
end
|
|
end
|
|
return lua
|
|
|
|
compile [action %actions %body] to
|
|
lua> ".."
|
|
local lua = \(compile as: local action %actions %body)
|
|
lua:remove_free_vars(table.map(\%actions, function(a) return "A"..string.as_lua_id(a.stub) end))
|
|
return lua
|
|
|
|
compile [action %action] to
|
|
Lua value "A\(%action.stub as lua id)"
|
|
|
|
compile [parse %actions as %body] to
|
|
lua> ".."
|
|
local replacements = {}
|
|
for i,arg in ipairs(\%actions[1]:get_args()) do
|
|
replacements[arg[1]] = tostring(nomsu:compile(arg))
|
|
end
|
|
local function make_tree(t)
|
|
if not AST.is_syntax_tree(t) then
|
|
return repr(t)
|
|
elseif t.type ~= 'Var' then
|
|
local args = {repr(tostring(t.source)), unpack(table.map(t, make_tree))}
|
|
return t.type.."("..table.concat(args, ", ")..")"
|
|
elseif replacements[t[1]] then
|
|
return replacements[t[1]]
|
|
else
|
|
return t.type.."("..repr(tostring(t.source))..", "..repr(t[1].." \\0").."..string.format('%X', __MANGLE_INDEX))"
|
|
end
|
|
end
|
|
local \%new_body = LuaCode(\%body.source,
|
|
"__MANGLE_INDEX = (__MANGLE_INDEX or 0) + 1",
|
|
"\nlocal tree = ", make_tree(\%body),
|
|
"\nlocal lua = nomsu:compile(tree); return lua")
|
|
local ret = \(compile as: compile %actions to %new_body)
|
|
return ret
|
|
|
|
compile [%tree as lua expr] to
|
|
Lua value "nomsu:compile(\(=lua "nomsu:compile(\%tree):as_expr()")):as_expr()"
|
|
|
|
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
compile [%tree as lua] to
|
|
Lua value "nomsu:compile(\(%tree as lua expr))"
|
|
|
|
compile [%tree as lua statements] to
|
|
Lua value "nomsu:compile(\(%tree as lua expr)):as_statements()"
|
|
|
|
compile [%tree as lua return] to
|
|
Lua value "nomsu:compile(\(%tree as lua expr)):as_statements('return ')"
|
|
|
|
compile [remove action %action] to
|
|
Lua ".."
|
|
A\(=lua "string.as_lua_id(\(%action.stub))") = nil
|
|
|
|
compile [%tree as nomsu] to
|
|
Lua value "nomsu:tree_to_nomsu(\(%tree as lua expr))"
|
|
|
|
compile [%tree as inline nomsu] to
|
|
Lua value "nomsu:tree_to_nomsu(\(%tree as lua expr), true)"
|
|
|
|
action [%var as lua identifier, %var as lua id]
|
|
lua> ".."
|
|
if type(\%var) == 'string' then return string.as_lua_id(\%var)
|
|
elseif \%var.type == 'Var' then return string.as_lua_id(\%var[1])
|
|
elseif \%var.type == 'Action' then return "A"..string.as_lua_id(\%var.stub)
|
|
end
|
|
|
|
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
|
|
compile [% is syntax tree] to
|
|
Lua value "AST.is_syntax_tree(\(% as lua expr))"
|
|
|
|
compile [% is %kind syntax tree] to
|
|
Lua value "AST.is_syntax_tree(\(% as lua expr), \(%kind as lua expr))"
|
|
|
|
compile [%tree with %t -> %replacement] to
|
|
Lua value ".."
|
|
\(%tree as lua expr):map(function(\(%t as lua expr))
|
|
\(%replacement as lua return)
|
|
end)
|
|
|
|
compile [%tree with vars %v] to
|
|
Lua value ".."
|
|
\(%tree as lua expr):map(function(t)
|
|
local replacements = \(%v as lua expr)
|
|
if t.type == "Var" then
|
|
return replacements[t[1]]
|
|
end
|
|
end)
|
|
|
|
compile [declare locals in %code] to
|
|
Lua value "\(%code as lua expr):declare_locals()"
|
|
|
|
compile [declare locals %locals in %code] to
|
|
Lua value "\(%code as lua expr):declare_locals(\(%locals as lua expr))"
|
|
|
|
compile [add free vars %vars to %code] to
|
|
Lua "\(%code as lua expr):add_free_vars(\(%vars as lua expr));"
|
|
|
|
compile [remove free vars %vars from %code] to
|
|
Lua "\(%code as lua expr):remove_free_vars(\(%vars as lua expr));"
|
|
|
|
compile [%lua <-write %code, to %lua write %code] to: Lua "\(%lua as lua expr):append(\(%code as lua expr));"
|
|
|
|
compile [to %lua write %code joined by %glue] to: Lua "\(%lua as lua expr):concat_append(\(%code as lua expr), \(%glue as lua expr));"
|
|
|
|
compile [quote %s] to
|
|
Lua value ".."
|
|
repr(\(%s as lua expr))
|
|
compile [type of %obj] to: Lua value "type(\(%obj as lua expr))"
|
|
|
|
compile [parse %text] to
|
|
Lua value ".."
|
|
nomsu:parse(NomsuCode("\("\(%text.source)")", \(%text as lua expr)))
|
|
|
|
compile [parse %text from %filename] to
|
|
Lua value ".."
|
|
nomsu:parse(NomsuCode(Source(\(%filename as lua expr), 1, #\(%text as lua expr)), \(%text as lua expr)))
|
|
|
|
compile [run %nomsu_code] to
|
|
Lua value "nomsu:run(\(%nomsu_code as lua expr), \(=lua "repr(tostring(\(%nomsu_code.source)))"))"
|
|
|
|
action [run tree %tree, %tree as value]
|
|
lua> ".."
|
|
return nomsu:run(\%tree)
|
|
|
|
compile [compile %block, compiled %block, %block compiled] to
|
|
Lua value "nomsu:compile(\(%block as lua))"
|
|
|
|
# Return statement is wrapped in a do..end block because Lua is unhappy if you
|
|
put code after a return statement, unless you wrap it in a block.
|
|
compile [return] to: Lua "do return; end"
|
|
compile [return %return_value] to: Lua "do return \(%return_value as lua expr) end"
|
|
|
|
# Literals
|
|
compile [yes] to: Lua value "true"
|
|
compile [no] to: Lua value "false"
|
|
compile [nothing, nil, null] to: Lua value "nil"
|
|
compile [Nomsu syntax version] to: Lua value "NOMSU_SYNTAX_VERSION"
|
|
compile [Nomsu compiler version] to: Lua value "NOMSU_COMPILER_VERSION"
|
|
compile [core version] to: Lua value "NOMSU_CORE_VERSION"
|
|
compile [lib version] to: Lua value "NOMSU_LIB_VERSION"
|
|
compile [command line args] to: Lua value "arg"
|
|
|
|
~~~~
|
|
compile [with local compile actions %body] to
|
|
Lua ".."
|
|
do
|
|
local nomsu = table.fork(nomsu, {COMPILE_ACTIONS=table.fork(nomsu.COMPILE_ACTIONS)})
|
|
\(%body as lua statements)
|
|
end
|
|
|
|
action [Nomsu version]
|
|
use "lib/version.nom"
|
|
return "\(Nomsu syntax version).\(core version).\(Nomsu compiler version).\(lib version)"
|